How to Create Buttons using Bootstrap
You can apply button classes for hyperlinks (<a>), button elements (<button>), and inputs (<input>). You need to add class .btn to make a link or element look like a button. Bootstrap provides many options to style buttons.
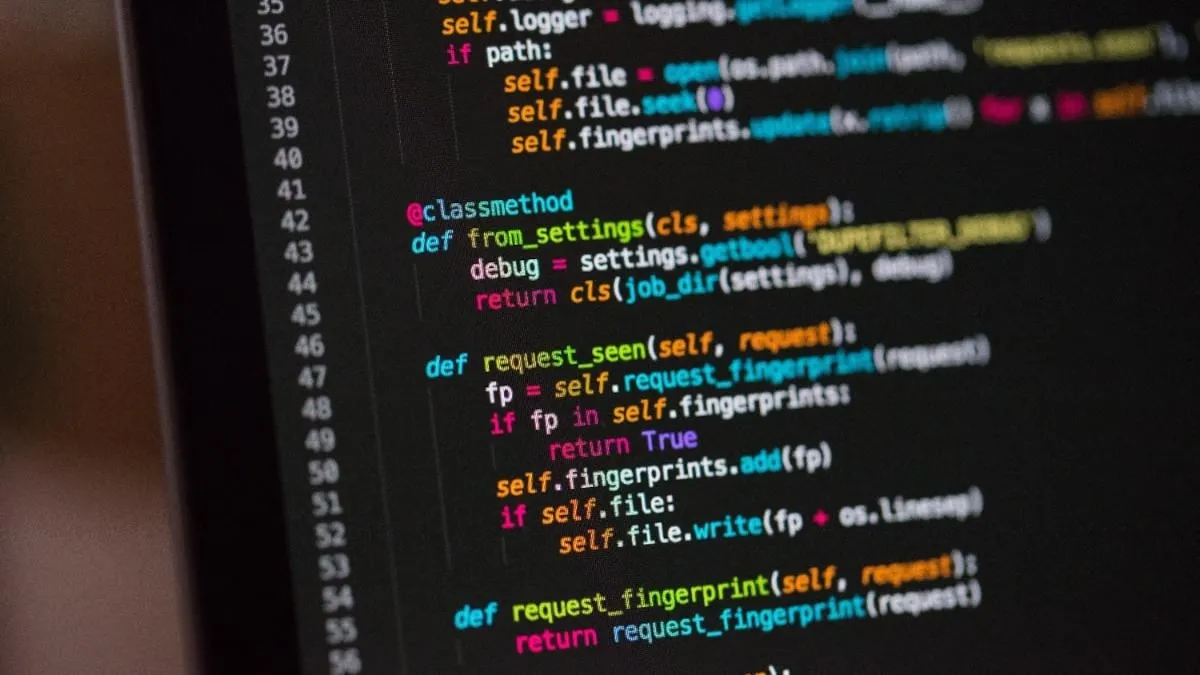
For example,
<button type="button" class="btn btn-primary">Primary</button>
When using button classes on <a> elements that are used to trigger in-page functionality (like collapsing content), rather than linking to new pages or sections within the current page, these links should be given a role="button" to appropriately convey their purpose to assisstive technologies such as screen readers. For example,
<a class="btn btn-primary" href="#" role="button">Link</a>
Contextual Colors and Styles
- .btn-default
- .btn-primary
- .btn-success
- .btn-info
- .btn-warning
- .btn-danger
- .btn-light
- .btn-dark
- .btn-link
1. Outline Buttons
You can remove the background colors on button by replacing the class with the .btn-outline-*. For example,
<button type="button" class="btn btn-outline-warning">Warning</button>
2. Button Sizes
You can add .btn-lg or .btn-sm for additional sizes. For example,
<button type="button" class="btn btn-secondary btn-lg">Large button</button>
Bootstrap provides five button sizes:
- .btn-lg
- .btn-md
- .btn-sm
- .btn-xs
- .btn-block
You can also make a button span the full width of the parent element with the .btn-block class, changing the display of the button to block. For example,
<button type="button" class="btn btn-primary btn-lg btn-block">Block level button</button>
3. Button State: Active or Disabled
Buttons appear pressed (with a darker background, darker border, and inset shadow) when active. You can force the same active appearance with .active class. Also, include the aria-pressed="true" attribute. For example,
<a href="#" class="btn btn-primary btn-lg active" role="button" aria-pressed="true">Primary link</a>
You can make buttons look inactive by adding the disabled boolean attribute to any <button> element.
<button type="button" class="btn btn-secondary btn-lg" disabled>Button</button>
Disabled buttons using the <a> element behave a bit different:
For <a> elements, as they don’t support the disabled attribute, you have to add the .disabled class to make it visually appear disabled. Also, include the aria-disabled="true" attribute to indicate the state of the element. For example,
<a href="#" class="btn btn-primary btn-lg disabled" tabindex="-1" role="button" aria-disabled="true">Primary link</a>
Sometimes, keyboard navigation remains unaffected, meaning that sighted keyboard users and users of assistive technologies can still be able to activate these links. So, to be safe, add a tabindex="-1" attribute on these links to prevent them from receiving keyboard focus and use custom JavaScript to disable their functionality.
4. Button Groups
Button group is a series of buttons together on a single line. You can wrap a series of buttons with .btn-group class. For example,
<div class="btn-group" role="group" aria-label="Basic example">
<button type="button" class="btn btn-secondary">Left</button>
<button type="button" class="btn btn-secondary">Middle</button>
<button type="button" class="btn btn-secondary">Right</button>
</div>
In order for assistive technologies (such as screen readers) to convey that a series of buttons is grouped, an appropriate role attribute needs to be provided. For button groups, this would be role="group", while toolbars should have a role="toolbar". In addition, groups and toolbars should be given an explicit label.
Sizing: Instead of applying button sizing classes to every button in a group, just add .btn-group-* to each .btn-group, including each one when nesting multiple groups. For example,
<div class="btn-group btn-group-lg" role="group" aria-label="...">...</div>
Vertical: You can make a set of buttons appear vertically stacked rather than horizontally by using class .btn-group-vertical. For example,
<div class="btn-group-vertical">
...
</div>
5. Button Toolbar
You can combine sets of button groups into button toolbars with the class .btn-toolbar. For example,
<div class="btn-toolbar" role="toolbar" aria-label="Toolbar with button groups">
<div class="btn-group mr-2" role="group" aria-label="First group">
<button type="button" class="btn btn-secondary">1</button>
<button type="button" class="btn btn-secondary">2</button>
<button type="button" class="btn btn-secondary">3</button>
<button type="button" class="btn btn-secondary">4</button>
</div>
<div class="btn-group mr-2" role="group" aria-label="Second group">
<button type="button" class="btn btn-secondary">5</button>
<button type="button" class="btn btn-secondary">6</button>
<button type="button" class="btn btn-secondary">7</button>
</div>
<div class="btn-group" role="group" aria-label="Third group">
<button type="button" class="btn btn-secondary">8</button>
</div>
</div>