Bootstrap Grid System
The basis of the Bootstrap framework relies in its grid system. Bootstrap offers components that allow to structure a website in a responsive grid layout.
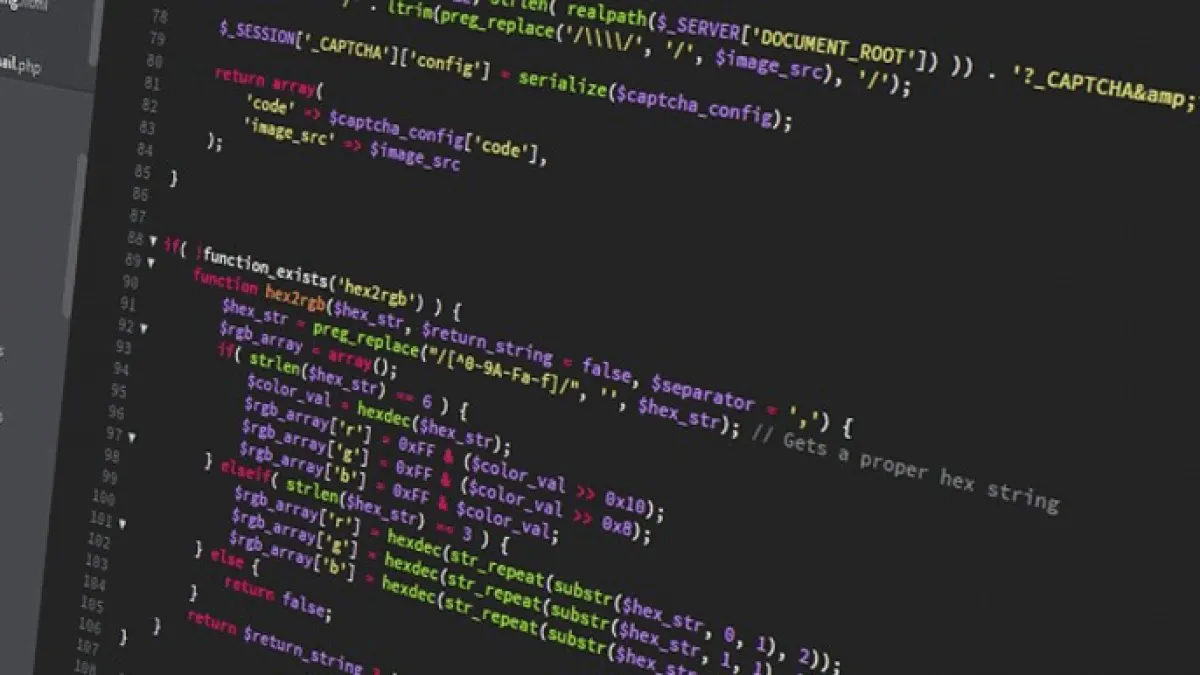
Bootstrap includes a responsive, mobile-first flexbox grid system to build layouts of all shapes and sizes. It appropriately scales up to 12 columns as the device or viewport size increases.
A viewport is the available display size to render the contents of a page. For example, the size of browser window, minus the toolbars, scrollbars, and so on. The viewport meta tag allows you to define the viewport's display size.
Mobile First Strategy: The code for Bootstrap starts by targeting smaller screens like mobile devices, tablets, and then expands for larger screens such as laptops, desktops.
Columns: Bootstrap's grid system allows up to 12 columns across the page. You can group the columns together to create wider columns.
How it Works?
Bootstrap's grid system uses a series of containers, rows, and columns to layout and align content. The grid is a structure that consists of three parts - an all encapsulating container, split into horizontal rows which are themselves split into 12 equal columns.
First, rows are placed within a .container (fixed-width) or .container-fluid (full-width) for proper alignment (center) and padding (15px on left and right).
<div class="container">
Then, use rows to create horizontal groups of columns. Every row is subdivided into 12 parts, so you can have up to 12 columns in a single row.
<div class="row">
Then, content is placed within columns. Columns are immediate children of rows.
Two Equal Column Layout
A basic grid system that creates two equal-width columns across all devices and viewports.
<div class="container">
<h1>Hello World!</h1>
<div class="row">
<div class="col">
<p>First Column</p>
</div>
<div class="col">
<p>Second Column</p>
</div>
</div>
</div>
Bootstrap Grid System
1. Containers
Containers are at the core of Bootstrap's grid system. It encapsulates all other content within a section of a page, providing the base for how the section is rendered.
Containers provide a means to center and horizontally pad your site's contents. A container can apply to the entire content of a page, where you would have one root container element, or to different sections of a page, where you would have numerous container elements on the page.
2. Rows
Rows are horizontal groups of columns that ensure columns are lined up properly. Use .row to ensure all the content is aligned properly down the left side. The power of the row lies in being able to stack content vertically.
3. Columns
Rows exist within containers, and those rows are split up into 12 equal columns. Content is placed within columns, and only columns are immediate children of rows.
Grid columns without a set width automatically have equal widths. For example, four instances of .col-sm will each automatically be 25% wide for small breakpoints.
Column classes indicate the number of columns you want to use out of the possible 12 per row. So, if you want three equal-width columns across, you can use .col-4. Columns have horizontal padding to create the gutters between individual columns.
4. Gutters
Gutters are the padding between columns, used to responsively space and align content in the Bootstrap grid system. Gutters provide horizontal (left and right) padding.
The .gx-* classes can be used to control the horizontal gutter widths. The .gy-* classes can be used to control the vertical gutter widths within a row when columns wrap to new lines. The .g-* classes can be used to control the horizontal and vertical gutter widths. You can apply these classes to the div element for row.