How to Create Forms using Bootstrap
A form contains many fields (also known as form controls) with optional labels and submit button. You should use appropriate type attribute on all inputs (email for email address or number for numerical information) to take advantage of newer input controls like email verification, number selection, and more.
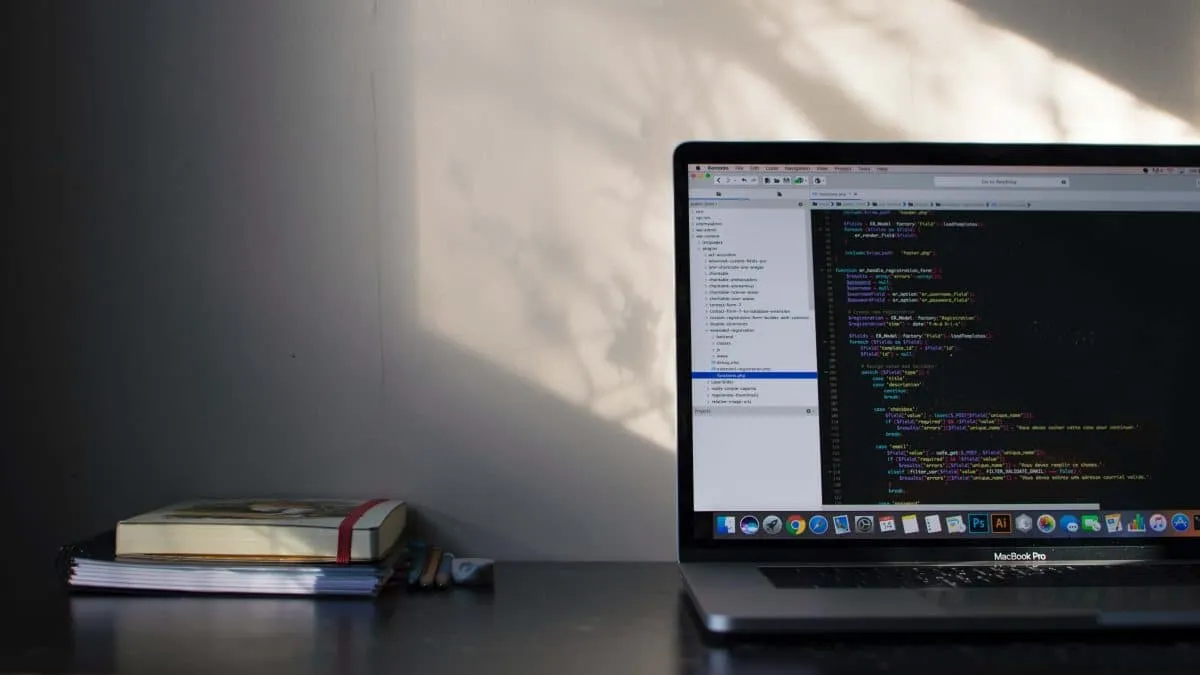
To create a basic form, you need to add only class: form-control on <input> and <textarea> elements.
Additionally, along with input field, each field can also contain label and help text. All these three should be in div block with bottom margin.
<form>
<div class="mb-3">
<label for="email" class="form-label">Email address</label>
<input type="email" class="form-control" id="email" aria-describedby="emailHelp">
<div id="emailHelp" class="form-text">We'll never share your email with anyone else.</div>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password">
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
Form text
Block-level or inline-level form text can be created using form-text class. Form text should be explicitly associated with the form control it relates to using the aria-describedby attribute. This will ensure that assistive technologies, such as screen readers, will announce this form text when the user focuses or enters the control.
Form label
Add class form-label to the label for the field.
Input Groups
You can add text, buttons, or button groups on either side of textual inputs, custom selects, and custom file inputs. This group with the <input> field is called input group.
You have to place the <label> element outside the input group.
<div class="input-group mb-3">
<span class="input-group-text" id="username">@</span>
<input type="text" class="form-control" placeholder="Username" aria-describedby="username">
</div>
Add the input-group class to the div block which will contain the input and text. Then, add input-group-text class to the span element for the text.
Floating Labels
You can create simple form labels that float over the input fields.
Add the class form-floating to the div block to enable floating labels with Bootstrap’s textual form fields.
<div class="form-floating mb-3">
<input type="email" class="form-control" id="floatingInput" placeholder="name@example.com">
<label for="floatingInput">Email address</label>
</div>
A placeholder is required on each <input> element. Also, the <input> must come before the <label>.