How To Redirect To Different URL in PHP
PHP How ToIf you want all the visitor on the webpage https://example.com/initial.php to redirect to https://example.com/final.php, this can be done using several methods using PHP, JavaScript and HTML.
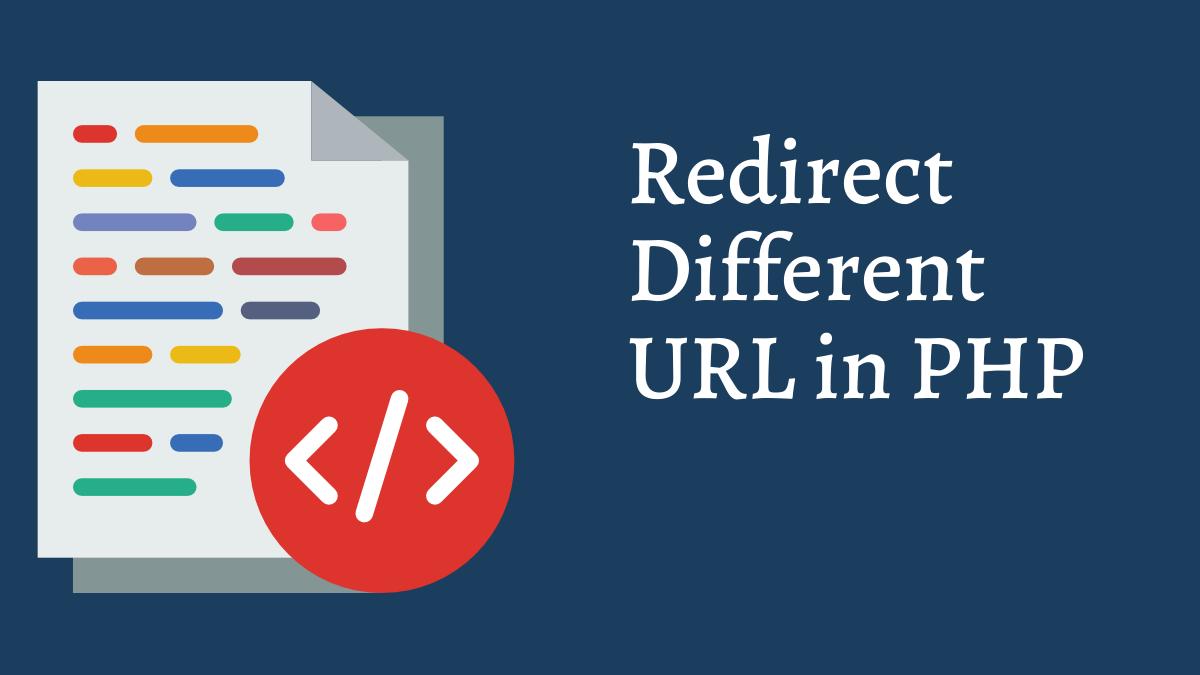
Using PHP header() Function to Redirect a URL
If you want to redirect initial.php to final.php, you can put the following code inside the initial.php webpage. This code sends a new HTTP location header to the browser.
$new_url = 'https://example.com/final.php';
header('Location: '.$new_url);
The above code should be placed before any HTML or text output. Otherwise, you will get an headers already sent error. You can also use output buffering in order to get rid of the headers already sent error.
ob_start();
$new_url = 'https://example.com/final.php';
header('Location: '.$new_url);
ob_end_flush();
The ob_start() function should be the first function in your PHP script when you want to perform a redirect using the header() function in PHP. This will prevent the headers already sent error from occurring.
As a safety measure, you might want to add die() or exit() directly after the header redirect in order to prevent the rest of the code of the webpage from executing.
$new_url = 'https://example.com/final.php';
header('Location: '.$new_url);
exit();
The die() or exit() have nothing to do with the redirection. They are used to prevent the rest of the code on the webpage from executing.
It is recommended to use absolute URLs while specifying a Location header value. However, relative URLs will also work just fine. You can also use this function to redirect users to external websites or webpages.