Defining a Class
A class is a blueprint of an object. What data an object should contain and what methods are needed to access that data can be described using a class. A class acts as a template for object creation.
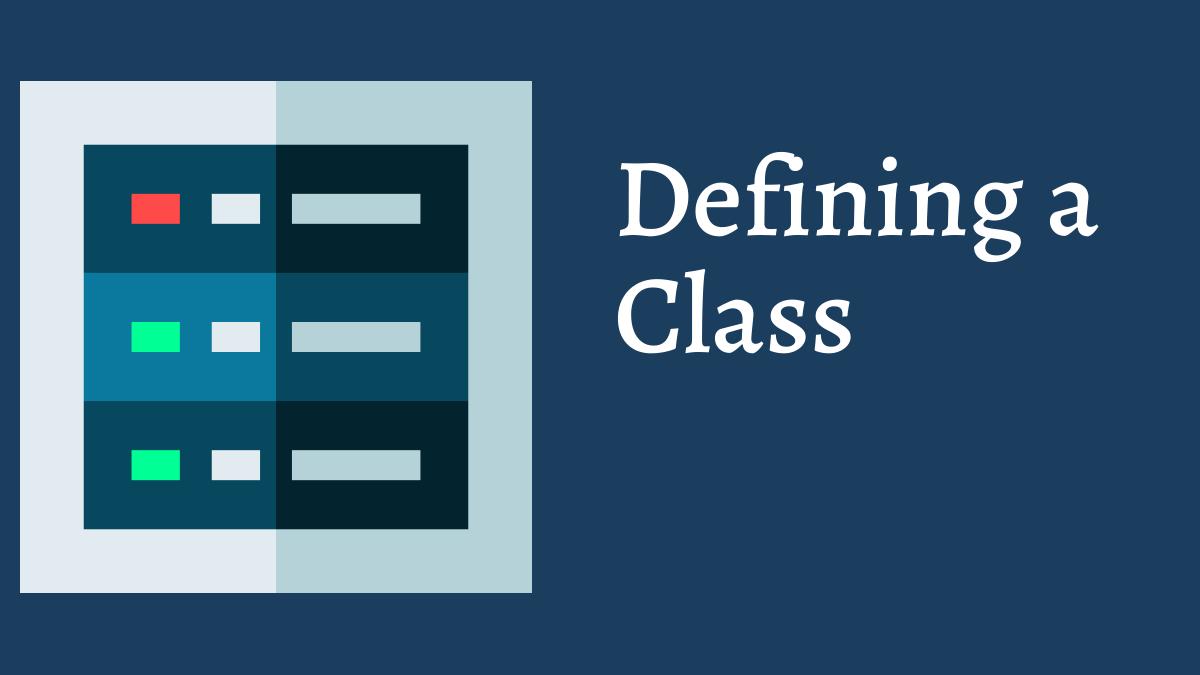
A class begins with the class keyword followed by the given name and the body enclosed in a pair of curly braces.
class className
{
Statements that define the properties
All the methods
}
The class body can contain properties and methods. All the property settings and method definitions are enclosed in the opening and closing curly braces. Properties are variables that hold the state of the object, whereas methods are functions that define what the object can do. In PHP, they need to have an explicit access level specified.
Example
class Person
{
public $name = 'John Doe';
function sayHello()
{
echo 'Hello!';
}
}
You can use any valid PHP identifier for the class name, except the name stdClass. PHP uses the name stdClass internally, so you can’t use this name.
If you want a class to be a subclass that inherits properties and methods, use a statement similar to the following:
class whiteRose extends Rose
{
Add the property statements
Add the methods
}
The object created from this class has access to all the properties and methods of both the whiteRose child class and the Rose class. The Rose class, however, doesn’t have access to properties or methods in the child class, whiteRose.
Class Properties
When you are defining a class, you declare all the properties at the top of the class. Properties are like variables inside the class. You can use these variables in objects.
class Car
{
private $color;
private $tires;
private $gas;
Method statements
}
Each property declaration begins with a keyword that specifies how the property can be accessed. The three keywords are:
- public: The property can be accessed from outside the class, either by the script or from another class.
- private: No access is granted from outside the class, either by the script or from another class.
- protected: No access is granted from outside the class except from a class that’s a child of the class with the protected property or method.
Classes should be written so that methods are used to access properties. By declaring a property to be private, you make sure that the property can’t be accessed directly from the script.
To set or change a variable’s value when you create an object, use the constructor.
Accessing Properties
Inside a class, $this is a special variable that refers to the properties of the same class. The $this can't be used outside of a class. It is designed to be used in statements inside a class to access variables inside the same class.
$this->varname;
A dollar sign ($) appears before this but not before variable name.
Class Methods
Methods are functions defined inside a class. Like functions, methods get some arguments and perform some actions, optionally returning a value. The advantage of methods is that they can use the properties of the object that invoked them. Thus, calling the same method in two different objects might have two different results.
Methods define what an object can do and are written in the class in the same format you’d use to write a function.
class CustomerOrder
{
private $total = 0;
function addItem($amount)
{
$this->total = $this->total + $amount;
echo "$amount was added; current total is $this->total";
}
}
Like functions, methods accept values passed to them.
The $this variable represents the object itself, and allows you to access the properties and methods of that same object.
Methods can be declared public, private, or protected, just as properties can. Public is the default access method if no keyword is specified.
PHP provides some special methods with names that begin with __ (two underscores). PHP handles these methods differently internally.
Instantiating a Class
The class code needs to be in the script that uses the class. Most commonly, the class is stored in a separate file and is included in any script that uses the class.
To use an object, you first create the object from the class. Then, that object can perform any methods that the class includes. Creating an object is called instantiating the object. You can use a class to create many similar but individual objects. To create an object, use statements that have the following format:
$object = new className(value, value);
The object is stored in the variable name.
Accessing Object Members
To access members that belong to an object, the single arrow operator (->) is needed. It can be used to call methods or to assign values to properties.
$object->propertyName;
$object->methodName();
Object creation involves memory allocation followed by the constructor method being called automatically.
The $this Variable
The $this is the pseudo variable that is available when class member variables or methods are called within an object context. $this works when you have instantiated a class and can be used to access the corresponding object's members.
So, to access an attribute in an object context, use $this->attribute_name, and to access a method, use $this->methodName().
Conventions for Classes
When working with classes, you should know that there are some conventions that everyone tries to follow in order to ensure clean code which is easy to maintain. The most important ones are as follows:
- Each class should be in a file named the same as the class along with the .php extension.
- Class names should be in CamelCase, that is, each word should start with an uppercase letter, followed by the rest of the word in lowercase.
- A file should contain only the code of one class.
- Inside a class, you should first place the properties, then the constructor, and finally, the rest of the methods.