PHP Namespacing
You cannot have two classes with the same name, since PHP would not know which one is being referred to when creating a new object. To solve this issue, PHP allows the use of namespaces, which act as paths in a file system. In this way, you can have as many classes with the same name as you need, as long as they are all defined in different namespaces.
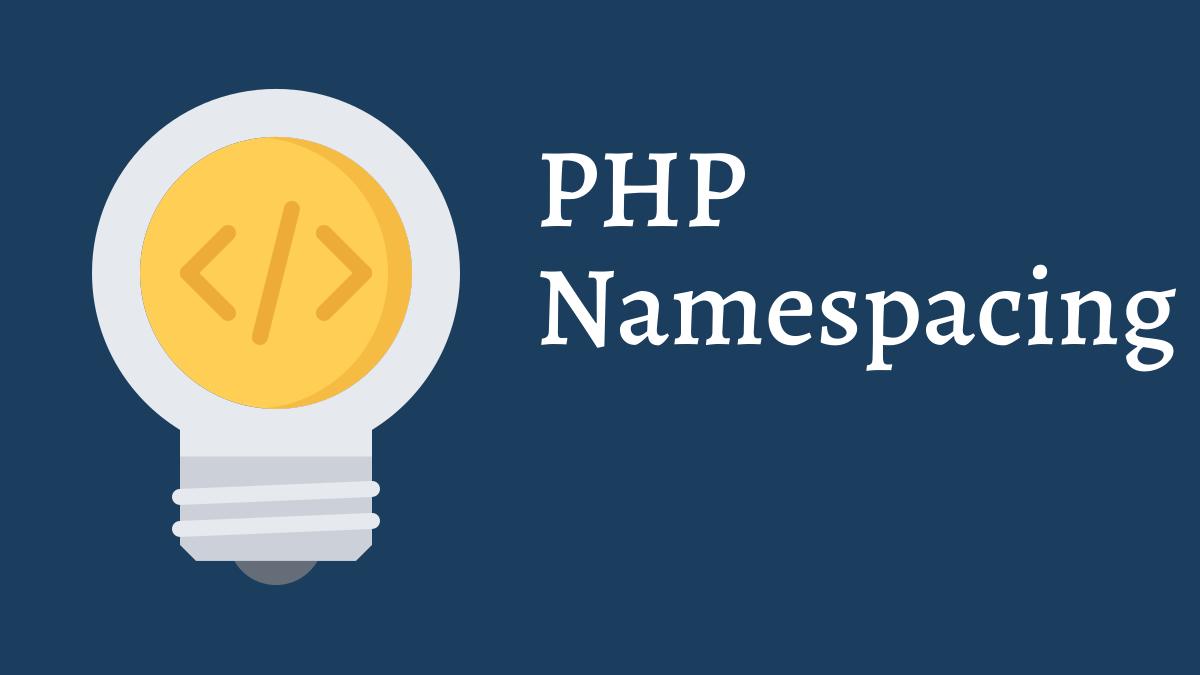
Specifying a namespace has to be the first thing that you do in a file. In order to do that, use the namespace keyword followed by the namespace. Each section of the namespace is separated by \, as if it was a different directory. If you do not specify the namespace, the class will belong to the base namespace, or root.
Namespaces are like a virtual directory structure for the classes. They were introduced from the PHP 5.3 version.
Why Use Namespaces
Namespaces solve two main problems:
- Name collisions between your code, and internal PHP classes, functions or constants or third-party classes, functions, or constants.
- Ability to alias (or shorten) Extra_Long_Names designed to alleviate the first problem, improving readability of source code.
As your PHP code library increases, it will be more likely you will accidentally reuse a function or class name that has been declared before. The problem is increased when you attempt to add third-party components or plugins. What if two or more code sets use same class name?
To solve this problem, long class or function names are being used. This worked decently, and there were even auto loading standards that separated the class names for folders on the file system. But, it was pretty messy, and often ended up with very long class names.
Name collision problems can be solved with namespaces. PHP constants, classes, and functions can be grouped into namespaced libraries. Namespace allows you to use the same function or class name in different parts of the same program without causing a name collision.
Defining Namespaces
Namespaced code is defined using a single namespace keyword at the top of your PHP file. It must be the first command and no non-PHP code or white-space can precede the command.
<?php
// define this code in the MyProject namespace
namespace MyProject;
The code following this line will belong to the MyProject namespace. It is not possible to nest namespaces or define two or more namespaces for the same code block (only the last will be recognized).
Example
In the example, we are creating a new class called Earth inside of the Solar namespace.
<?php
namespace Solar;
class Earth
{
}
If you didn't use a namespace and you had another class with the name of 'Earth' included in your application you would get an error stating you cannot declare class again. With namespaces, you can create another class with the same name as:
<?php
namespace Planet;
class Earth
{
}
Now, you can easily distinguish which Earth class you want to use.
// create a new Earth in the Solar namespace
$earth1 = new Solar\Earth();
// create a new Earth in the Planet namespace
$earth2 = new Planet\Earth();
Usually when using namespaces, try to follow the folder structure of your namespaces so it's easier to find where these files are located. So our Solar/Earth.php file would be in a Solar folder.
Guideline
As the number of components involved in a web application grow, so too increases the potential for name clashes. One solution for this is to prefix names with the name of the component. However, this creates long names, which reduces readability of the source code. For this reason, PHP 5.3 introduced namespaces, which allow developers to group code for each component into separately named containers.