PHP Arrays
An array is used to store a collection of values in a single variable. Arrays in PHP consist of key-value pairs. The key can either be an integer (numeric array) or as a string (associative array). The value can be any data type.
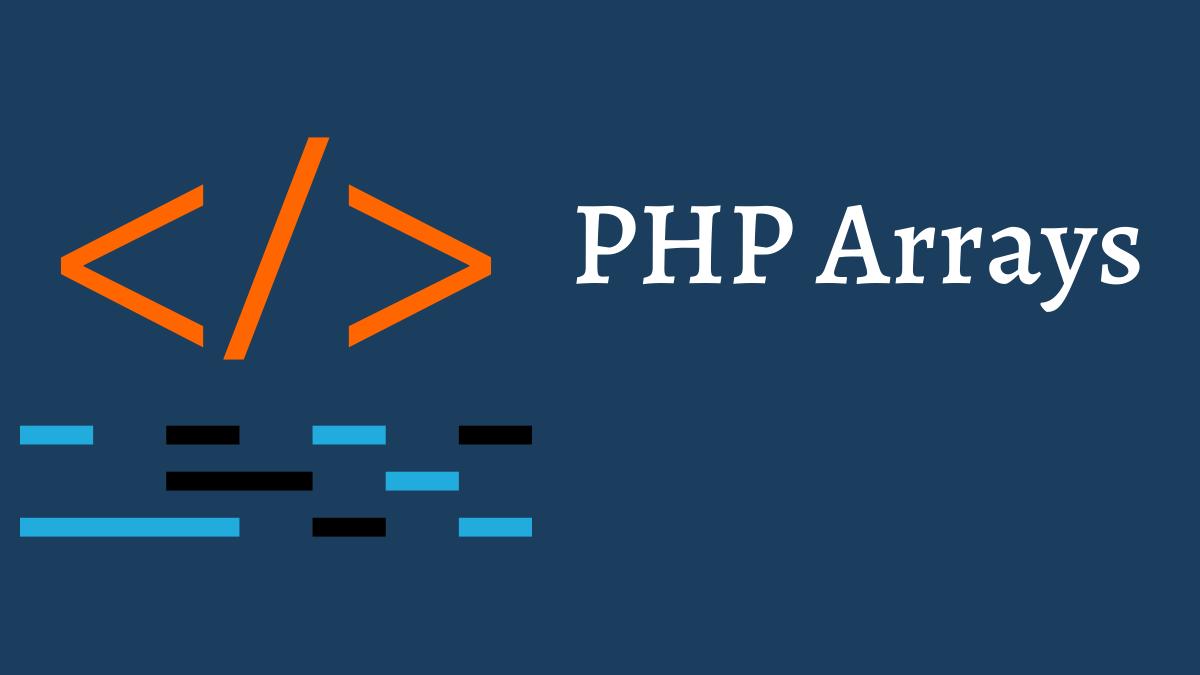
Arrays are complex variables. An array stores a group of values under a single variable name. It is useful for storing related values as a linked list of information. Information in an array can be handled, accessed, and modified easily.
1. Initializing or Creating Arrays
You have different options for initializing an array. You can initialize an empty array, or you can initialize an array with data. There are different ways of writing the same data with arrays too.
The simplest way to create an array is to assign a value to a variable with square brackets ([ ]) at the end of its name. For example, the following statement creates an array called $cities:
$cities[0] = "Phoenix";
At this point, the array named $cities has been created and has only one value.
$cities[1] = "Tucson";
$cities[2] = "Flagstaff";
Now the array $cities contains three values: Phoenix, Tucson, and Flagstaff.
$cities = array("Phoenix","Tucson","Flagstaff");
This statement also creates the same array.
$empty_array = array();
Keys of an array can be any alphanumeric value, like strings or numbers. Values of an array can be anything: strings, numbers, Booleans, other arrays, and so on.
$books = [
'1984' => [
'author' => 'George Orwell',
'finished' => true,
'rate' => 9.5
],
'Romeo and Juliet' => [
'author' => 'William Shakespeare',
'finished' => false
]
];
This array is a list that contains two arrays - maps. Each map contains different values like strings, doubles, and Booleans.
2. Removing Element from Array
If you need to remove an element from the array, instead of adding or updating one, you can use the unset function:
$status = [
'name' => 'James Potter',
'status' => 'alive'
];
unset($status['status']);
print_r ($status);
The new $status array contains the key name only.
3. Types of Arrays
There are two types of arrays:
- Numeric Indexed Array
- Associative Array
The difference is in the way the key is specified.
In a numeric indexed array, the keys are numeric and starts with 0, and the values can be any data type.
$a = array(1,2,3);
Once the array is created, its elements can be referenced by placing the index of the desired element in square brackets. The index begins with zero.
$a[0] = 1;
$a[1] = 2;
$a[2] = 3;
The number of elements in the array is handled automatically. Adding a new element to the array is as easy as assigning a value to it.
$a[3] = 4;
The index can also be left out to add the value to the end of the array. This syntax also constructs a new array if the variable does not already contain one.
$a[] = 5; // $a[4]
In an associative array, the keys are not necessarily numeric, and even when they are numeric, not necessarily in any order. So, when you put data into an associative array, you need to specify both the key and the value. When creating the array the double arrow operator (=>) is used to tell which key refers to what value.
$b = array('one' => 'a', 'two' => 'b', 'three' => 'c');
Elements in associative arrays are referenced using the element names. They cannot be referenced with a numeric index.
$b['one'] = 'a';
$b['two'] = 'b';
$b['three'] = 'c';
4. Multi-Dimensional Array
A multi-dimensional array is an array that contains other arrays. These are array of arrays. For example, a two-dimensional array can be constructed in the following way.
$a = array(array('00', '01'), array('10', '11'));
Once created, the elements can be modified using two sets of square brackets.
$a[0][0] = '00';
$a[0][1] = '01';
$a[1][0] = '10';
$a[1][1] = '11';
For example, suppose you want to store cities by state and county, as follows:
$cities[‘AZ’][‘Maricopa’] = Phoenix;
$cities[‘AZ’][‘Cochise’] = Tombstone;
$cities[‘AZ’][‘Yuma’] = Yuma;
$cities[‘OR’][‘Multnomah’] = Portland;
$cities[‘OR’][‘Tillamook’] = Tillamook;
$cities[‘OR’][‘Wallowa’] = Joseph;
$cities is a two-dimensional array.
You can walk through a multidimensional array by using foreach statements. You need a foreach statement for each array. One foreach statement is inside the other foreach statement. Putting statements inside other statements is called nesting.
foreach ($cities as $state)
{
foreach ($state as $county => $city)
{
echo “$city, $county county <br />”;
}
}
5. Sorting Arrays
One of the most useful features of arrays is that PHP can sort them for you. PHP originally stores array elements in the order in which you create them. If you display the entire array without changing the order, the elements will be displayed in the order in which you created them.
An array can be sorted in different ways, so there are a lot of chances that the order that you need is different from the current one. By default, the array is sorted by the order in which the elements were added to it, but you can sort an array by its key or by its value, both ascending and descending. Furthermore, when sorting an array by its values, you can choose to preserve their keys or to generate new ones as a list.
6. Accessing Arrays
Lists are treated internally as a map with numeric keys in order. The first key is always 0; so, an array with n elements will have keys from 0 to n-1. Trying to access a key that does not exist in an array will return you a null and throw a notice, as PHP identifies that you are doing something wrong in your code.
You will often want to do something to every value in an array. You might want to echo each value, store each value in the database, or add some number to each value in the array. Walking through each and every value in an array, in order, is called iteration or traversing.
The foreach statement walks through the array one value at a time.
foreach ($array_name as $key_name => $value_name)
{
block of statements;
}
- array_name is the name of the array that you are walking through.
- key_name is the name of the variable where you want to store the key. It is optional.
- value_name is the name of the variable where you want to store the value.
7. The empty and isset functions
There are two useful functions about the content of an array. If you want to know if an array contains any element at all, you can ask if it is empty with the empty function. That function actually works with strings too, an empty string being a string with no characters (' ').
The isset function takes an array position, and returns true or false depending on whether that position exists or not.
$string = '';
$array = [];
$names = ['Harry', 'Ron', 'Hermione'];
var_dump(empty($string)); // true
var_dump(empty($array)); // true
var_dump(empty($names)); // false
var_dump(isset($names[2])); // true
var_dump(isset($names[3])); // false
An array with no elements or a string with no characters will return true when asked if it is empty, and false otherwise.
8. Searching Elements in Array
One of the most used functions with arrays is in_array. This function takes two values, the value that you want to search for and the array. The function returns true if the value is in the array and false otherwise. This is very useful, because a lot of times what you want to know from a list or a map is if it contains an element, rather than knowing that it does or its location.
Even more useful sometimes is array_search. This function works in the same way except that instead of returning a Boolean, it returns the key where the value is found, or false otherwise.
$names = ['Harry', 'Ron', 'Hermione'];
$containsHermione = in_array('Hermione', $names);
var_dump($containsHermione); // true
$containsSnape = in_array('Snape', $names);
var_dump($containsSnape); // false
$wheresRon = array_search('Ron', $names);
var_dump($wheresRon); // 1
$wheresVoldemort = array_search('Voldemort', $names);
var_dump($wheresVoldemort); // false
9. print_r and var_dump
You can see the structure and values of any array by using a print_r or a var_dump statement. You can check the result from your browser by using the function print_r. It does something similar to var_dump, just without the type and size of each value.
When printing the content of an array, it is useful to see one key-value per line, but if you check your browser, you will see that it displays the whole array in one line. That happens because what the browser tries to display is HTML, and it ignores new lines or white spaces. To check the content of the array as PHP wants you to see it, check the source code of the page - you will see the option by right-clicking on the page.