How to Create Image using PHP
PHP How ToMost of the image based operations can be performed by using PHP built-in functions. The image-based PHP functions are in GD library and you can use them for ctraeting an image.
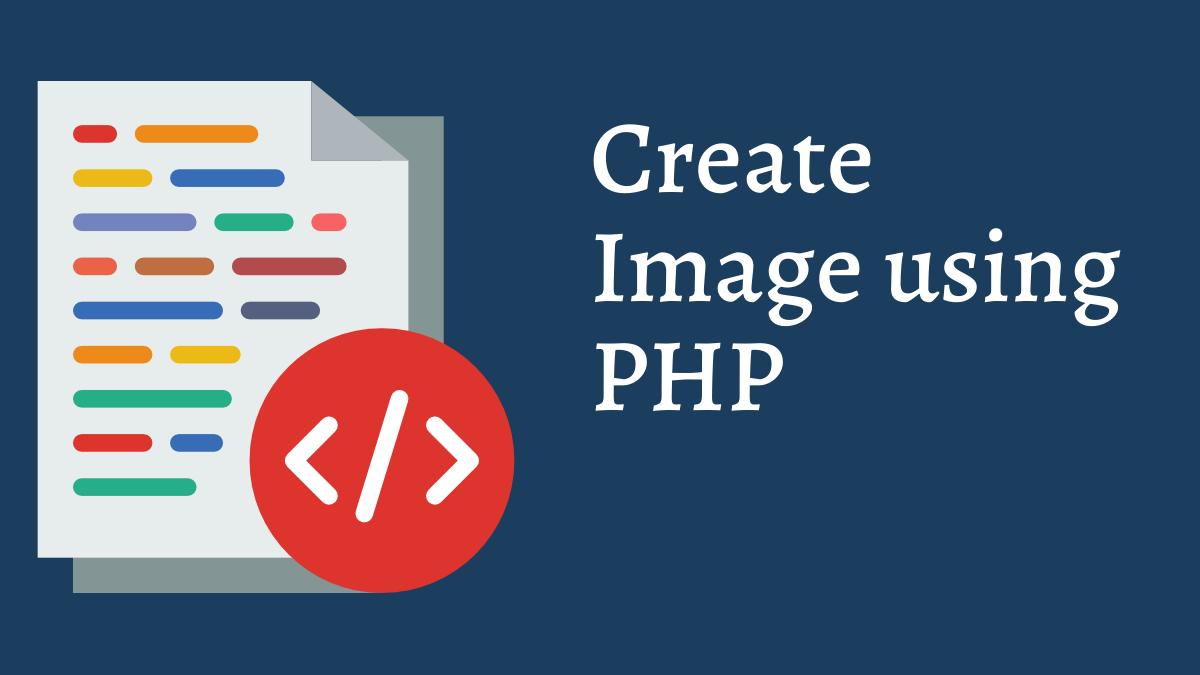
Step 1
The imagecreate() function is used to create a new palette based image. It returns an image identifier representing a blank image of specified size.
You can also use imagecreatetruecolor() function to create a new true color image. It returns an image identifier representing a black image of the specified size.
For example:
$img = imagecreate($width, $height);
Step 2
Next, use imagecolorallocate() function to allocate a color for an image. It returns a color identifier representing the color composed of the given RGB components. The values of RGB parameters are integers between 0 and 255 or hexadecimals between 0x00 and 0xFF.
The first call to imagecolorallocate() fills the background color in palette-based images - images created using imagecreate().
$background_color = imagecolorallocate($img, 0, 0, 0);
$text_color = imagecolorallocate($img, 233, 14, 91);
Step 3
You can use the imagestring() function to draw a string horizontally at the given coordinates.
imagestring($img, $font, $x, $y, $text, $text_color);
The $font can be 1, 2, 3, 4, or 5 for built-in fonts in latin2 encoding (higher numbers corresponds to larger fonts). You can use your own font identifiers registered with imageloadfont().
The $x is x-coordinate of the upper left corner. The $y is y-coordinate of the upper left corner. The $text is string to be written.
Step 4
Finally, use imagepng() function to output a PNG image to either the browser or a file.
// Output Image
header('Content-type: image/png');
imagepng($img);
imagedestroy($img);
// Or save Image
imagepng($img, "test.png");
Modify Existing Image
In many cases, you usually design the basic background of your image and only add any additional text or graphical elements that need to be dynamically drawn using PHP.
To use an existing GIF, JPEG or PNG image as a canvas on which you add additional elements, use one of the following functions instead of imagecreate().
- imagecreatefromgif ($filename)
- imagecreatefromjpeg ($filename)
- imagecreatefrompng ($filename)