How to Create Table in SQL
The CREATE TABLE statement is used to create a new table in a database. The column specifies the name of the column of the table. The datatype parameter specifies the type of data the column can hold.
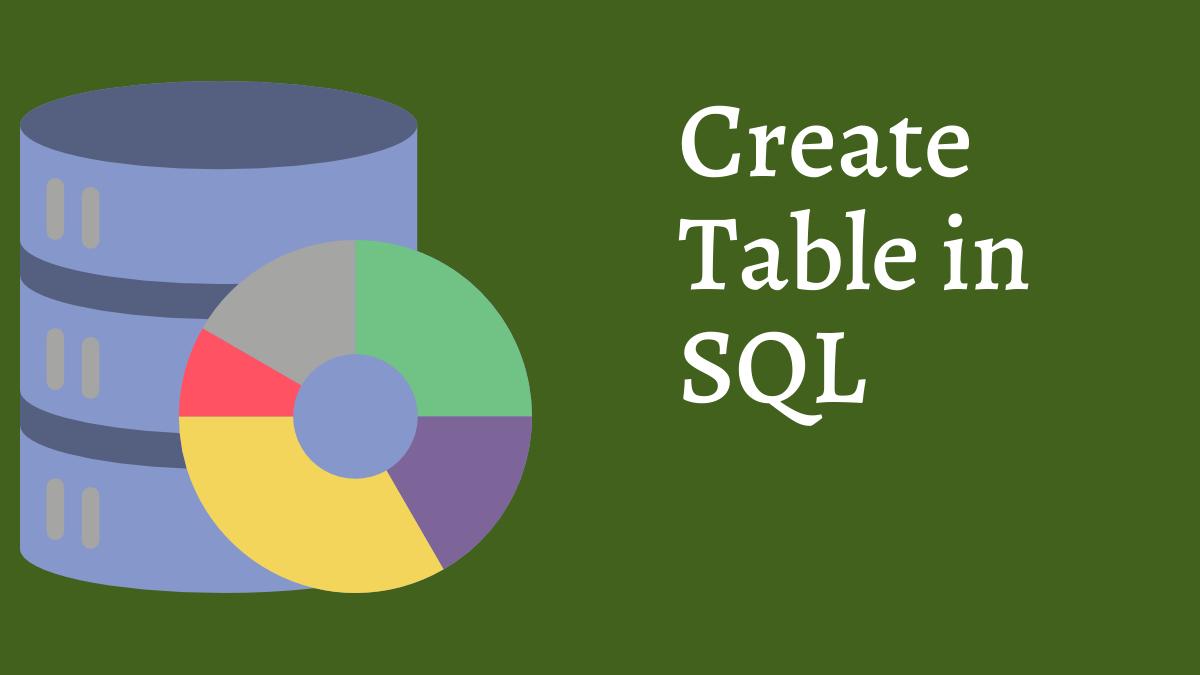
CREATE TABLE table_name (
column1 datatype,
column2 datatype,
column3 datatype,
....
);
1. Creating Table with Constraints
Constraints can be specified when the table is created with the CREATE TABLE statement, or after the table is created with the ALTER TABLE statement. Constraints are used to specify rules for the data in a table.
CREATE TABLE table_name (
column1 datatype constraint,
column2 datatype constraint,
column3 datatype constraint,
....
);
Constraints are used to limit the type of data that can go into a table. This ensures the accuracy and reliability of the data in the table. If there is any violation between the constraint and the data action, the action is aborted.
For example,
CREATE TABLE employees (
id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT,
firstname VARCHAR(25) NOT NULL,
lastname VARCHAR(25) NOT NULL,
email VARCHAR(45) NOT NULL,
phone VARCHAR(10) NOT NULL,
PRIMARY KEY(id));
The following constraints are used in SQL:
- NOT NULL: Ensures that a column cannot have a NULL value
- UNIQUE: Ensures that all values in a column are different
- PRIMARY KEY: A combination of a NOT NULL and UNIQUE. Uniquely identifies each row in a table
- FOREIGN KEY: Uniquely identifies a row or record in another table
- CHECK: Ensures that all values in a column satisfies a specific condition
- DEFAULT: Sets a default value for a column when no value is specified
- INDEX: Used to create and retrieve data from the database very quickly
NOT NULL
Although SQL allows a column to contain null values, there are times when you want to be sure that a column always has a distinct value. In order for one row in a table to be distinguished from another, there must be some way of telling them apart. This is usually done with a primary key, which must have a unique value in every row. Because a null value in a column could be anything, it might match the value for that column in any of the other rows. Thus it makes sense to disallow a null value in the column that is used to distinguish one row from the rest.
UNIQUE
The NOT NULL constraint is a fairly weak constraint. You can satisfy the constraint as long as you put anything at all into the field. The UNIQUE constraint will not only disallow the entry of a null value in a column, but it will also disallow the entry of a value that matches a value already in the column.
CHECK
Use the CHECK constraint for preventing the entry of invalid data that goes beyond maintaining uniqueness. For example, you can check to make sure that a numeric value falls within an allowed range. You can also check to see that a particular character string is not entered into a column.
2. Conditionally Creating a Table
By default, MySQL generates an error if you attempt to create a table that already exists. To avoid this error, the CREATE TABLE statement offers a clause that can be included if you want to simply abort the table-creation attempt if the target table already exists. For example,
CREATE TABLE IF NOT EXISTS employees (
id TINYINT UNSIGNED NOT NULL AUTO_INCREMENT,
firstname VARCHAR(25) NOT NULL,
lastname VARCHAR(25) NOT NULL,
email VARCHAR(45) NOT NULL,
phone VARCHAR(10) NOT NULL,
PRIMARY KEY(id));
3. Copying a Table
The following query produces an exact copy of the employees table, naming it employees2:
CREATE TABLE employees2 SELECT * FROM employees;
Sometimes you need to create a table based on just a few columns found in a preexisting table. You can do so by simply specifying the columns within the CREATE SELECT statement:
CREATE TABLE employees3 SELECT firstname, lastname FROM employees;
4. Viewing Available Tables
You can view a list of the tables available to a database with the SHOW TABLES statement.
5. Viewing a Table Structure
You can view a table structure using the DESCRIBE statement. Alternatively, you can use the SHOW command to produce the same result.
SHOW columns IN employees;
6. Deleting a Table
Deleting a table, or dropping it, is accomplished via the DROP TABLE statement.
DROP TABLE employees;
You could also simultaneously drop employees2 and employees3 tables like:
DROP TABLE employees2, employees3;