CSS Media Queries for Responsive Web Design
A media query consists of a media type and zero or more expressions that check for the conditions of particular media features. Without the CSS3 media query, you would be unable to target particular CSS styles at particular device capabilities, such as the viewport width.
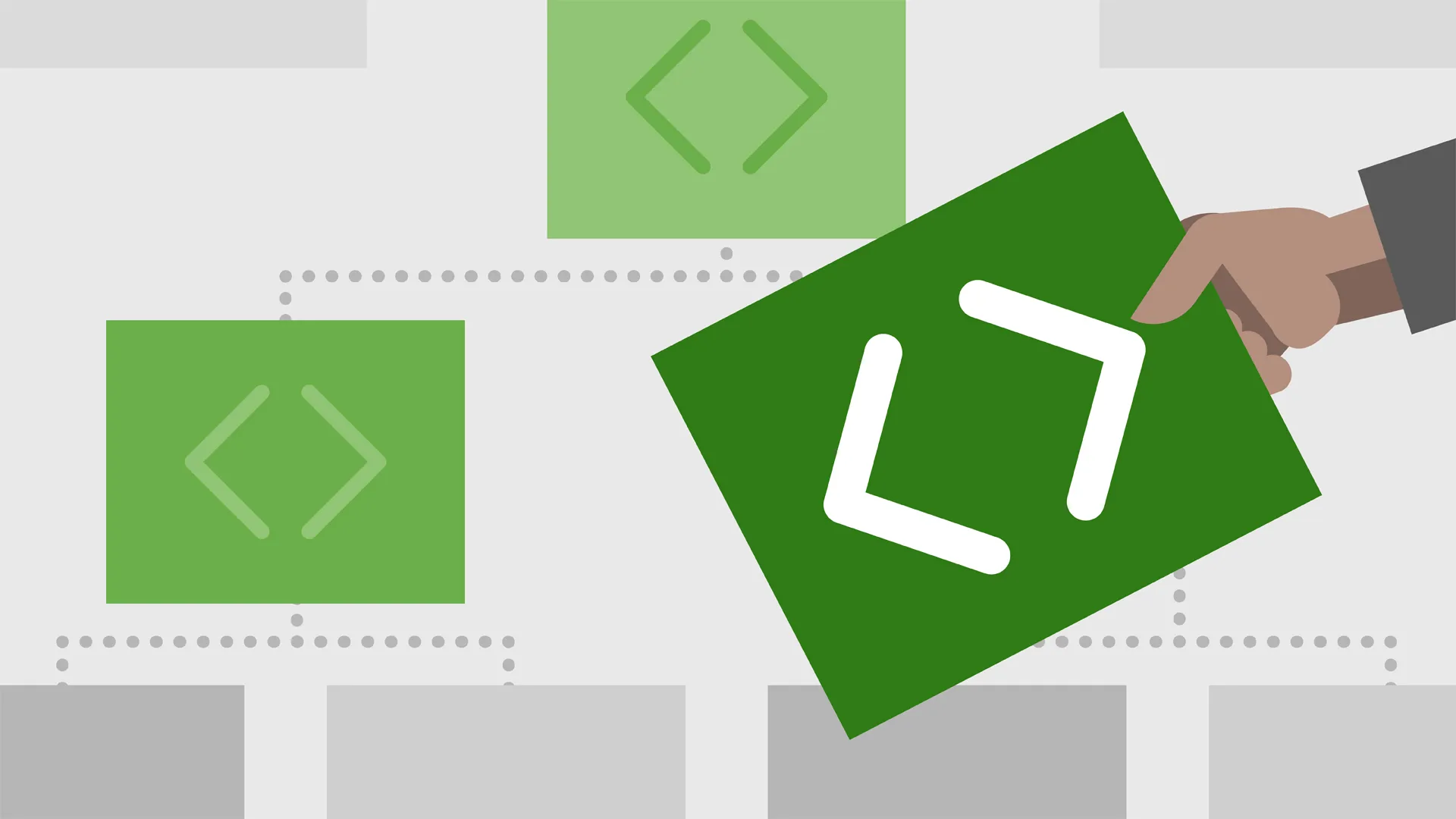
For example, the background color of the page will vary depending upon the current viewport size.
body {
background-color: grey;
}
@media screen and (max-width: 960px) {
body {
background-color: red;
}
}
@media screen and (max-width: 768px) {
body {
background-color: orange;
}
}
@media screen and (max-width: 550px) {
body {
background-color: yellow;
}
}
@media screen and (max-width: 320px) {
body {
background-color: green;
}
}
Fixed Width Design
A very simple and common structure includes header, navigation, sidebar, content, and footer. The header and footer are 940 pixels wide (with 10-pixels margin on either side), and the sidebar and content occupy 220 and 700 pixels, respectively, with a 10-pixel margin on either side of each.
#wrapper {
margin-right: auto;
margin-left: auto;
width: 960px;
}
#header {
margin-right: 10px;
margin-left: 10px;
width: 940px;
background-color: #779307;
}
#navigation ul li {
display: inline-block;
}
#sidebar {
margin-right: 10px;
margin-left: 10px;
float: left;
background-color: #fe9c00;
width: 220px;
}
#content {
margin-right: 10px;
float: right;
margin-left: 10px;
width: 700px;
background-color: #dedede;
}
#footer {
margin-right: 10px;
margin-left: 10px;
clear: both;
background-color: #663300;
width: 940px;
}
As the viewport decreases to less than 960 pixels, areas of the content at the right start getting clipped.
Stop Mobile Browsers from Auto-Resizing Page
You need to add the <meta> tag within the <head> tags of the HTML.
<meta name="viewport" content="width=device-width,initial-scale=1.0" />
The scale to 1.0 means that the mobile browser will render the page at 100 percent of its viewport. Setting the device's width means that the page should render at 100 percent of the width of all supported mobile browsers.
Now, the browsers will not zoom the page, so you can design for different viewports. In the CSS, you add a media query for devices such as tablets that have a viewport width of 768 pixels in portrait view.
@media screen and (max-width: 768px) {
#wrapper {
width: 768px;
}
#header,#footer,#navigation {
width: 748px;
}
#content,#sidebar {
padding-right: 10px;
padding-left: 10px;
width: 728px;
}
}
The media query is re-sizes the width of the wrapper, header, footer, and navigation elements if the viewport size is no larger than 768 pixels.
The Problem
Anything with a viewport under 768 pixels is going to experience clipping and anything between 768 and 960 pixels will experience clipping as it will get the non-media query version of the CSS styles.
At present, when you resize our viewport, the design snaps at the points that the media queries intervene and the shape of our layout changes. However, it then remains static until the next viewport "break point" is reached. The solution is to move from a rigid and fixed layout to a fluid layout.
Fluid Layouts
You need to set a proportional value for the width that the #wrapper should be in relation to the viewport size.
#wrapper {
margin-right: auto;
margin-left: auto;
width: 96%;
}
Grid View
A responsive grid-view often has 12 columns, and has a total width of 100%, and will shrink and expand as you resize the browser window.
First, all HTML elements should have the box-sizing property set to border-box. This makes sure that the padding and border are included in the total width and height of the elements. Add the following code in your CSS:
* {
box-sizing: border-box;
}
Now, calculate the percentage for one column as:
100% / 12 = 8.33%
Then, make one class for each of the 12 columns, class="col-" and a number defining how many columns the section should span. All these columns should be floating to the left, and have a padding of 15px.
[class*="col-"] {
float: left;
padding: 15px;
border: 1px solid red;
}
Each row should be wrapped in a <div>. The number of columns inside a row should always add up to 12. The columns inside a row are all floating to the left, and are therefore taken out of the flow of the page, and other elements will be placed as if the columns do not exist. To prevent this, add a style that clears the flow:
.row::after {
content: "";
clear: both;
display: table;
}
Media Queries
Add a breakpoint where certain parts of the design will behave differently on each side of the breakpoint. For example, when the screen gets smaller than 768px, each column should have a width of 100%.
Mobile First means designing for mobile before designing for desktop or any other device. Instead of changing styles when the width gets smaller than 768px, you should change the design when the width gets larger than 768px. This will make the design Mobile First.
[class*="col-"] {
width: 100%;
}
@media only screen and (min-width: 768px) {
/* For desktop: */
.col-1 {width: 8.33%;}
.col-2 {width: 16.66%;}
.col-3 {width: 25%;}
.col-4 {width: 33.33%;}
.col-5 {width: 41.66%;}
.col-6 {width: 50%;}
.col-7 {width: 58.33%;}
.col-8 {width: 66.66%;}
.col-9 {width: 75%;}
.col-10 {width: 83.33%;}
.col-11 {width: 91.66%;}
.col-12 {width: 100%;}
}
You can add as many breakpoints as you like.